When I first started creating mobile applications, I started with a silly game named Boun&Twee. At the time, Flutter didn't exist, so it was only compatible with Android. There was no animation, and updating the layout was painful with a lot of XML tweaking 😅
In this article, I'll try to share my learnings around flutter_animate
and how to use this library for Flutter animations.
Today with Flutter, writing such a small game is much more convenient. But nowadays, having a "fun" game isn't enough; you need animation everywhere! 🤪
At first, I wanted to use AnimationController manually, but it struck me: hasn't a new library for animation been announced during Flutter Vikings?
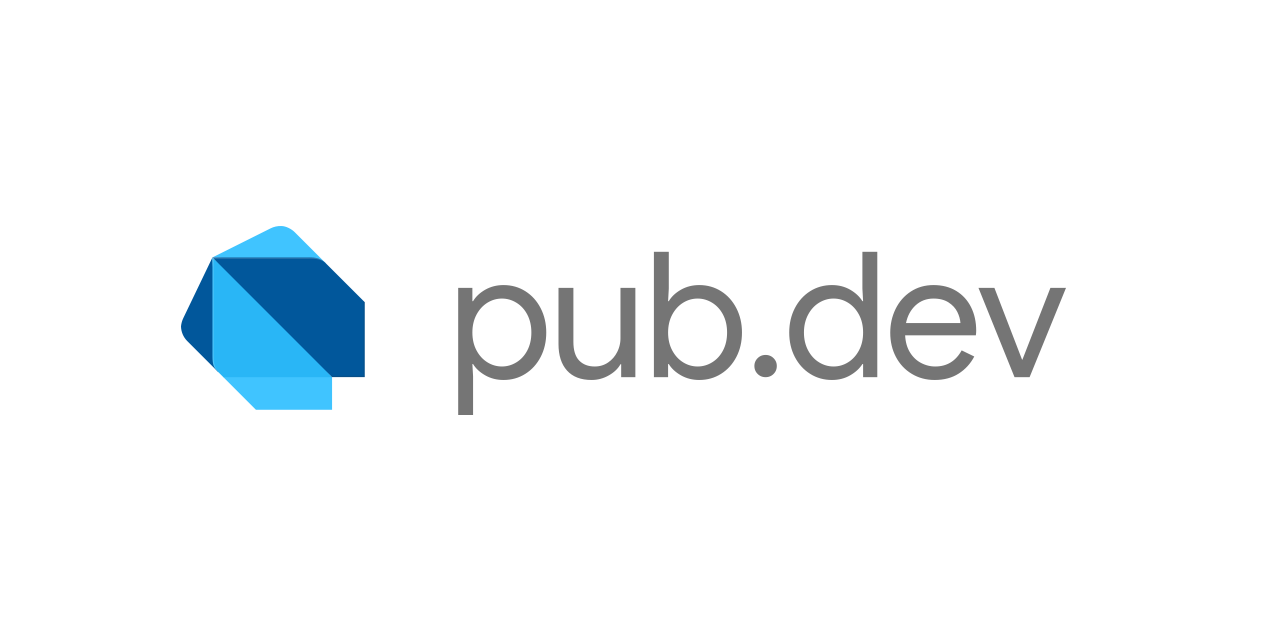
After a quick reading of the README, it appeared clear that flutter_animate
helps write much clearer code.
Let's try to write our first small animation.
The Game
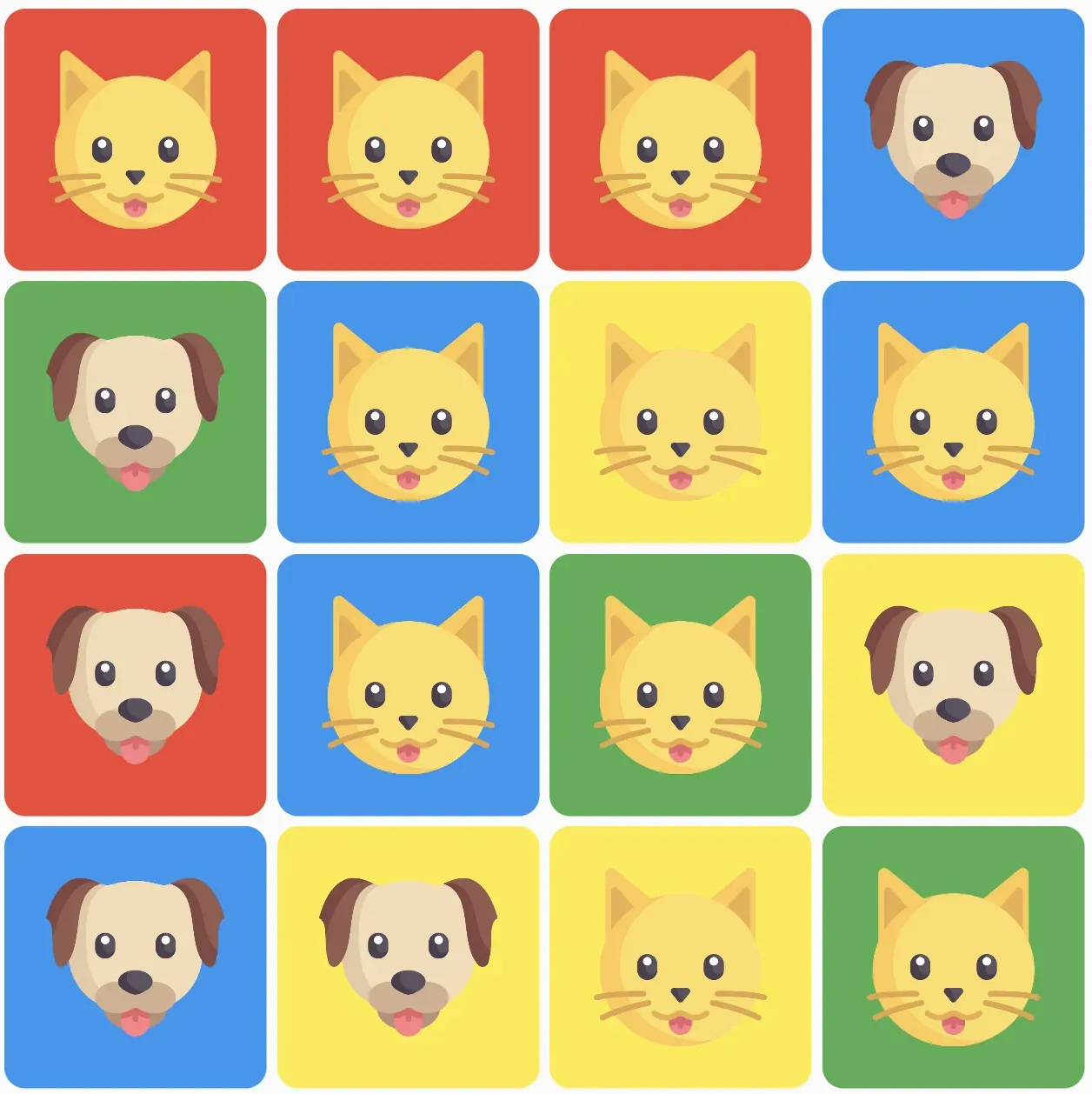
My game is pretty simple; you have two best friends in the world: a dog and a cat. You must pair a cat and a dog of the same color.
When you click simultaneously on a dog and a cat of the same color, the game shuffles, and you have to find a new pair. After 30 seconds, the game ends.
I've been playing with flutter_animate from @gskinner!
— BeGuillaume 💙 (@BeGuillaume) November 7, 2022
I'm impressed by how fast you can create some game animation with it!🚀
(still not using Flame though 😅) pic.twitter.com/zXGjyudbkA
The animation
I want to add a card flip animation to my game. Each card should flip when the board is shuffled to discover a new card.
Looking at the available animations in flutter_animate
you can find a FlipEffect
.
You can find below how I achieved this effect:
return Stack(
children: [
Container(
color: Colors.red,
width: 100,
height: 100,
).animate().flip(
direction: Axis.horizontal,
begin: 0,
end: 0.5,
duration: durationSpeed,
curve: Curves.easeInOut,
),
Container(
color: Colors.blue,
width: 100,
height: 100,
).animate().flip(
direction: Axis.horizontal,
begin: -0.5,
end: 0,
duration: durationSpeed,
delay: durationSpeed,
curve: Curves.easeInOut,
),
],
);
I've created a Stack
of widgets with two Container
. You then add a simple animate()
to be able to change animations. Here I've just added a flip
animation to each, with a delay for the second.
The other trick is to play with the value of begin
and end
values to have the second card flipped in the right direction.
Conclusion
As you can see, using flutter_animate
you can achieve an animation with a minimal amount of code. Having everything in a declarative way with your widget also helps with the lisibility of your animations. You also don't have to dispose of your controllers manually, which is always nice.
If you have any questions, don't hesitate to post a comment on the website or send me a message on Twitter, I'll be happy to help 😁